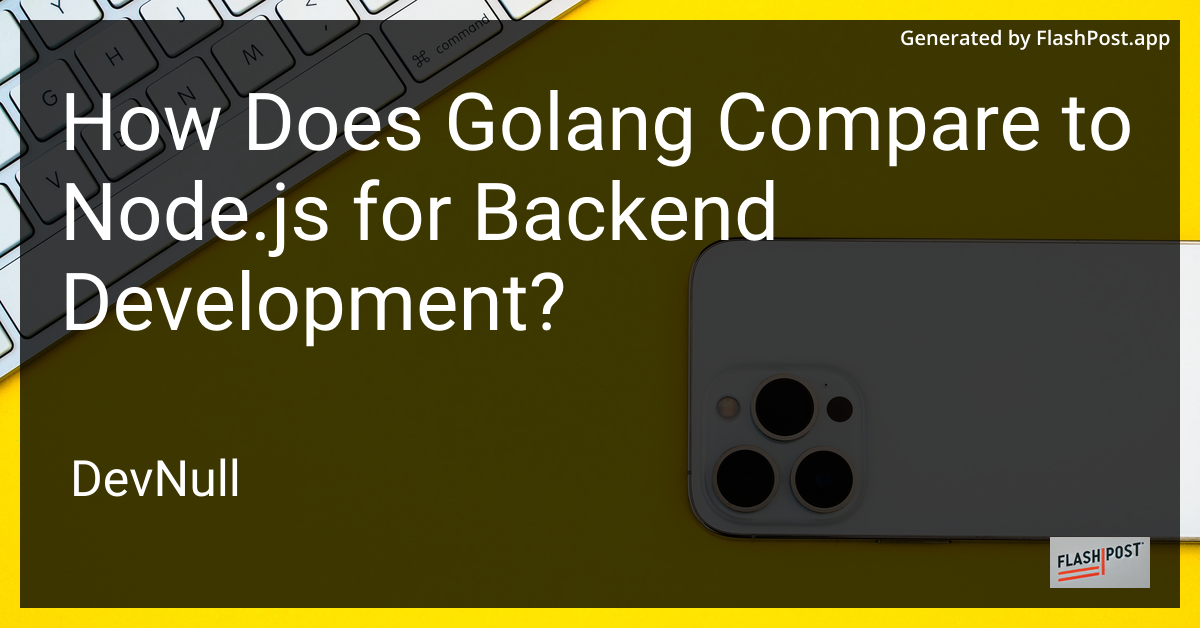
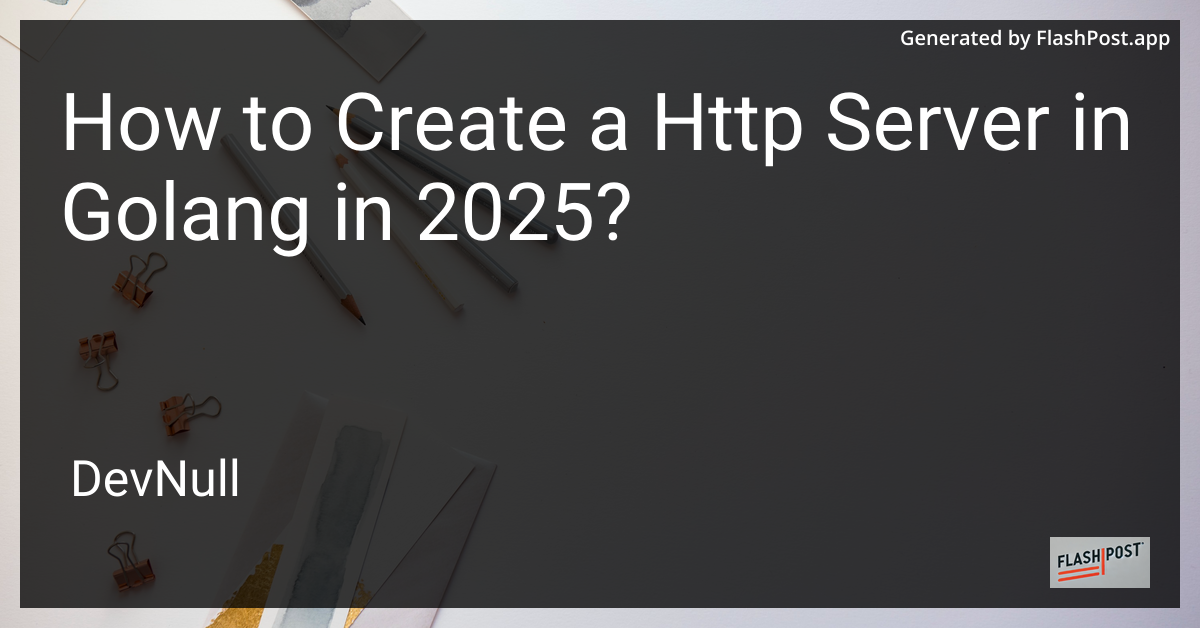
How to Create a Http Server in Golang in 2025?
In 2025, Golang continues to be one of the most prominent programming languages for building scalable and efficient web servers. Whether you’re developing a simple website or a complex API, understanding how to create an HTTP server in Golang is essential. In this article, we will walk you through the steps and provide some useful code examples to get you started.
Why Choose Golang for HTTP Servers?
Golang’s concurrency model, efficient performance, and simple syntax make it ideal for building HTTP servers. Its standard library offers robust packages that simplify many tasks involved in server creation.
Steps to Create an HTTP Server in Golang
Step 1: Setting Up Your Golang Environment
Before you start coding, ensure you have Go installed on your system. You can download it from the official Go website. Make sure to set up your GOPATH
and workspace directory properly.
Step 2: Writing a Simple HTTP Server
Start by creating a file, let’s say main.go
. Here’s a basic example of an HTTP server:
package main
import (
"fmt"
"net/http"
)
func handler(w http.ResponseWriter, r *http.Request) {
fmt.Fprintf(w, "Hello, Welcome to my HTTP server!")
}
func main() {
http.HandleFunc("/", handler)
fmt.Println("Starting server on :8080")
http.ListenAndServe(":8080", nil)
}
Step 3: Run Your Server
Navigate to the directory containing main.go
and run:
go run main.go
Visit http://localhost:8080
in your web browser, and you should see “Hello, Welcome to my HTTP server!” displayed on the page.
Step 4: Handling HTTP Requests
For more advanced request handling, including parsing query parameters and headers, you can extend the handler function. Learn more about Golang HTTP requests here.
Step 5: Error Handling
While building your server, robust error handling is critical. Golang provides various ways to compare and handle errors efficiently. You can find comprehensive details on comparing errors in Golang here.
Step 6: Deploying Your Golang HTTP Server
Once your server is ready and thoroughly tested, you’ll need to deploy it. Deployment strategies can vary depending on your infrastructure. Discover helpful tips for deploying a Golang application.
Best Practices for Building HTTP Servers in Golang
- Utilize Go’s Concurrency Features: Take advantage of Goroutines and Channels for handling multiple requests concurrently.
- Security Considerations: Always validate and sanitize user inputs to protect against common web vulnerabilities.
- Graceful Shutdown: Implement a mechanism for gracefully shutting down your server to ensure all requests are completed before termination.
Conclusion
Creating a simple HTTP server in Golang is straightforward with its powerful standard library and easy syntax. As you advance, you can leverage Golang’s features to build more complex and efficient servers. Remember to keep exploring and enhancing your server with additional functionality and best practices.
By following this guide, you will have a head start in building efficient HTTP servers with Golang in 2025. As technologies evolve, staying updated with the latest practices and resources remains a valuable asset.
Happy coding!