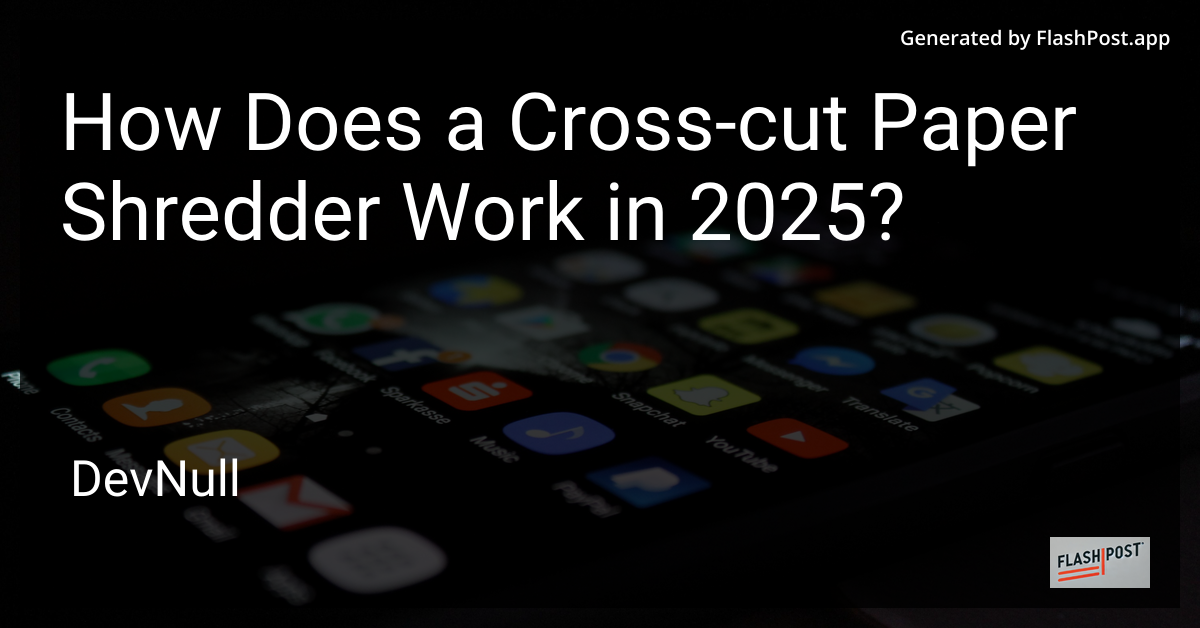
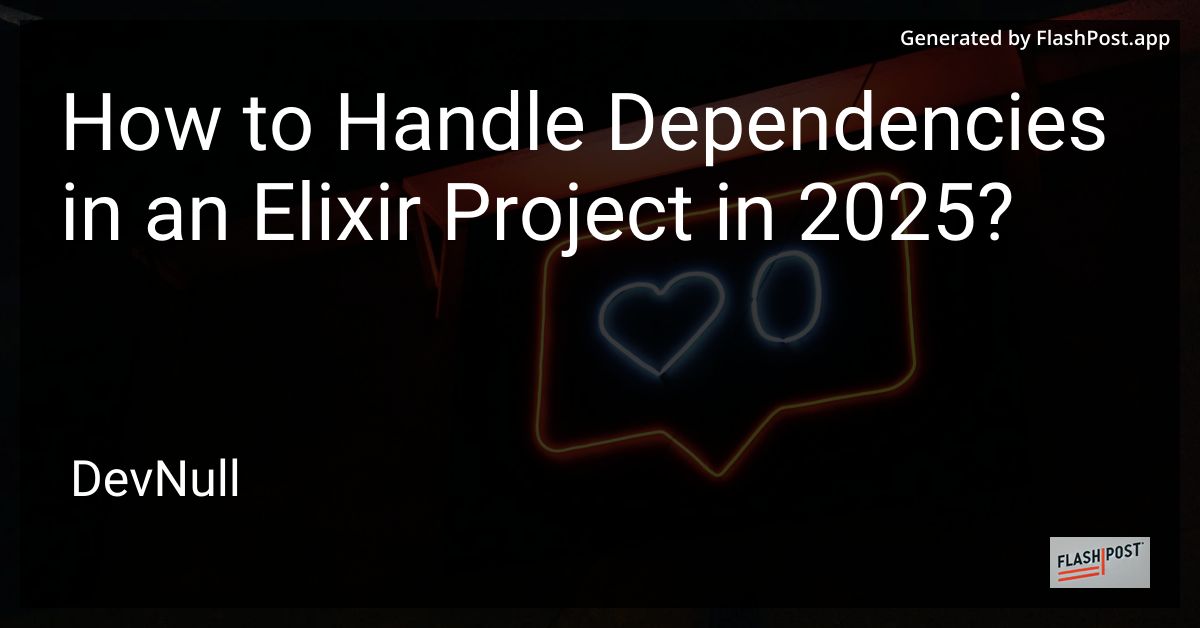
How to Handle Dependencies in an Elixir Project in 2025?
In the evolving landscape of software development, managing dependencies efficiently remains critical to ensure a seamless development process. As we step into 2025, handling dependencies in an Elixir project involves updated strategies and best practices that enhance maintainability and security. This guide provides a comprehensive look at handling dependencies in Elixir, leveraging the latest tools and techniques.
1. Understanding Mix and Hex
Elixir projects primarily use Mix and Hex to manage dependencies. Mix is a build tool that provides tasks for creating, compiling, testing Elixir projects, while Hex is the package manager crucial for handling libraries. Combining their capabilities makes dependency management streamlined.
Key Concepts:
-
Mix.exs File: The cornerstone for managing dependencies. This file contains all necessary configurations. Define your project’s dependencies in the
deps
function.defp deps do [ {:phoenix, "~> 1.6.0"}, {:ecto, "~> 3.8.0"} ] end
-
Hex.pm: The central repository that hosts a plethora of libraries, making importing and managing these libraries easy and secure.
For a deep dive into functional programming techniques, check out this exploration of how the pipe operator works in Elixir.
2. Verifying Integrity with Mix and Hex
Security is crucial when managing dependencies. Mix and Hex provide tools to verify the integrity of packages:
-
Package Checksums: When you fetch dependencies, Mix verifies the checksum of the downloaded packages to ensure integrity and security.
-
Lock File: The
mix.lock
file ensures that all dependencies are resolved in the same versions across different environments. Regularly update this file to reflect the latest compatible versions while maintaining stability.mix deps.get mix deps.update --all
3. Handling Environment-Specific Dependencies
In 2025, environment-specific dependency management is more sophisticated:
-
Conditional Dependencies: Use environment variables to define dependencies that are only needed in specific environments like test, dev, or prod.
defp deps do [ {:ex_machina, "~> 2.7", only: :test}, {:credo, "~> 1.6", only: [:dev, :test]} ] end
-
Configuring Per-Environment Settings: Customize configurations per environment to ensure that apps perform optimally regardless of the environment.
For insights into directory management in Elixir projects, explore how to create and use same directory names.
4. Leveraging Docker for Dependency Isolation
To maximize dependency management efficiency and prevent version clashes:
-
Docker Containers: Use Docker to create isolated environments for your Elixir applications. Containerizing your application ensures a consistent environment across development, testing, and production.
FROM elixir:1.14 WORKDIR /app COPY . . RUN mix deps.get
5. Regularly Auditing Dependencies
It’s vital to audit your dependencies to address vulnerabilities regularly:
-
Mix Audit: Utilize tools like
mix_audit
to automate the process of dependency checks. Incorporate auditing in CI/CD pipelines to catch issues early.mix audit
For further expansion on macro and advanced programming techniques, check this guide on expanding multiple macros in Elixir.
Conclusion
Handling dependencies in an Elixir project as of 2025 is all about utilizing robust tools like Mix and Hex while maintaining constant vigilance against security vulnerabilities. By incorporating environment-specific configurations, leveraging Docker, and performing regular audits, you can ensure your Elixir project’s dependencies are managed efficiently and securely. Whether you’re enhancing your project’s functional components or organizing its structure effectively, these strategies will help streamline your development process.