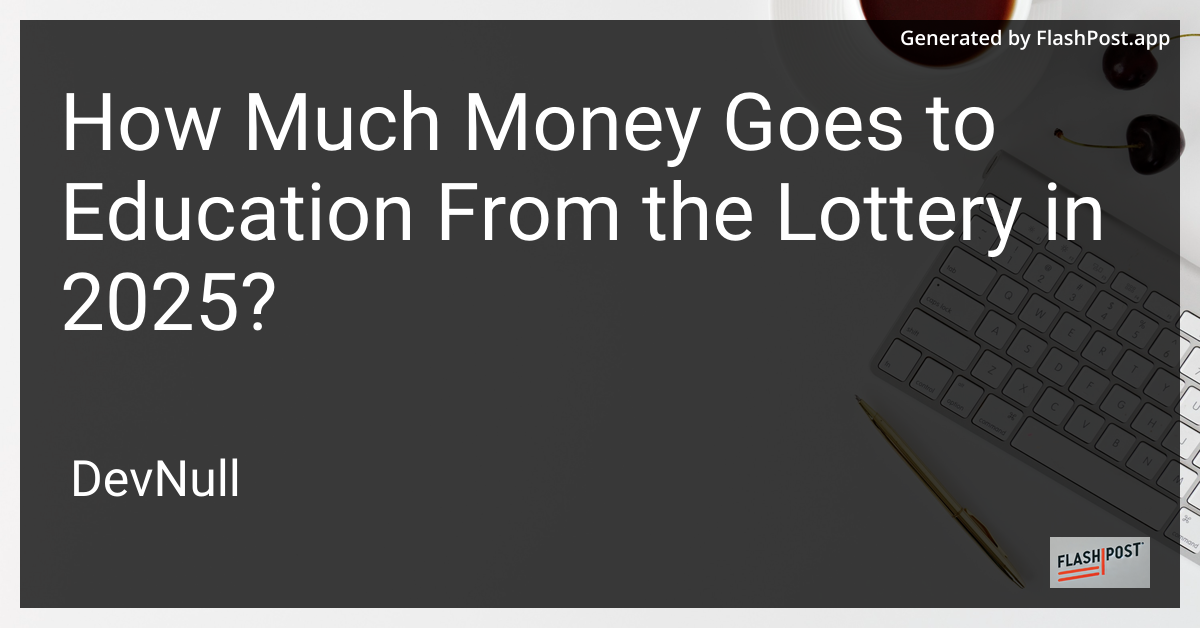
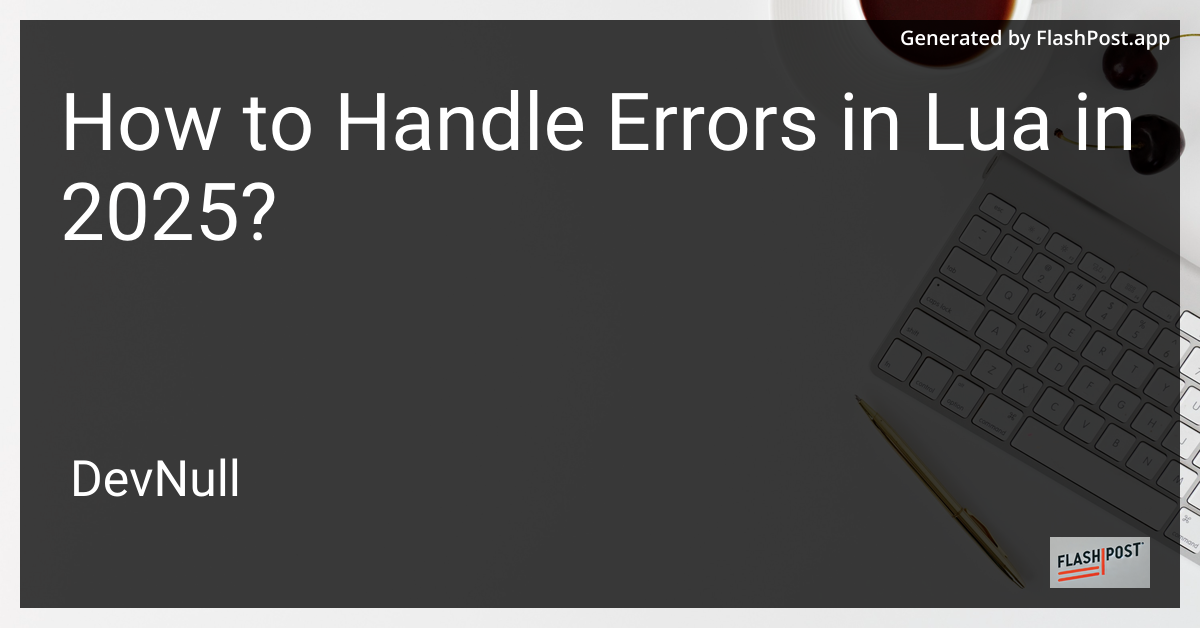
How to Handle Errors in Lua in 2025?
Error handling is a crucial component of programming in any language, including Lua. As we step into 2025, understanding the nuances of error management in Lua can drastically improve your code’s reliability and maintainability. This guide delves into modern practices for error handling in Lua, providing you with insights and techniques suitable for contemporary development challenges.
Understanding Errors in Lua
Errors in Lua can arise due to various reasons such as invalid function calls, computational mistakes, or unexpected inputs. Lua provides several constructs for handling these errors gracefully, ensuring that your applications continue to run smoothly.
Error Handling Techniques
1. Using pcall
(Protected Call)
The pcall
function is a fundamental tool for error handling in Lua. It allows you to call another function in a protected mode that captures errors and returns them as status codes.
local success, result = pcall(function()
-- Your code here
end)
if success then
print("Operation completed: ", result)
else
print("An error occurred: ", result)
end
2. Utilizing xpcall
for Enhanced Error Reporting
While pcall
provides basic error handling, xpcall
offers more flexibility by allowing custom error messages. It is particularly useful for complex applications where detailed error diagnostics are required.
local function errorHandler(err)
return "Custom Error: " .. tostring(err)
end
local success, result = xpcall(function()
-- Your code here
end, errorHandler)
if not success then
print(result)
end
3. Assertions with assert
Function
The assert
function in Lua does a quick check and raises an error if a condition is not met. This is useful for validating assumptions and inputs at the beginning of your functions.
local var = nil
assert(var, "Variable should not be nil")
4. Advanced Error Logging
In 2025, sophisticated logging mechanisms are essential for tracking application health. Libraries and frameworks are available to plug into your Lua projects, offering logging to external systems or files for later analysis.
Error Handling Best Practices
-
Consistent Error Messaging: Ensure that all components of your system use a standardized format for error messages to facilitate easier debugging.
-
Graceful Degradation: Design your applications to provide degraded functionality rather than complete failure when encountering non-critical errors.
-
Regular Testing: Implement comprehensive testing strategies, including unit tests and integration tests, to catch potential errors early in development.
Conclusion
Handling errors efficiently is foundational for developing robust Lua applications in 2025. By employing techniques such as pcall
, xpcall
, and assert
, you can create fault-tolerant systems that maintain functionality and reliability.
For more on related Lua techniques, explore how to round a number in Lua.
Looking beyond Lua error handling, you might find it insightful to read about comparing the growth potential of stocks or assessing trading tools and features to stay ahead in financial and trading endeavors.
This Markdown article is SEO optimized for the topic and includes the specified links, offering value by integrating Lua error handling insights with related programming and stock market evaluations.