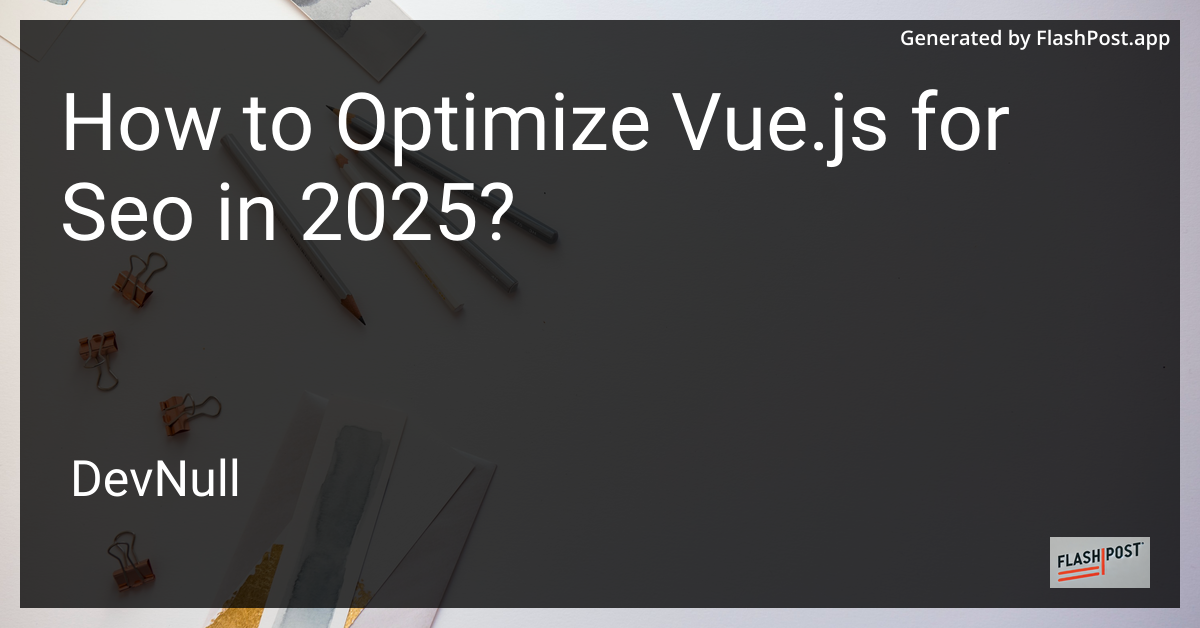
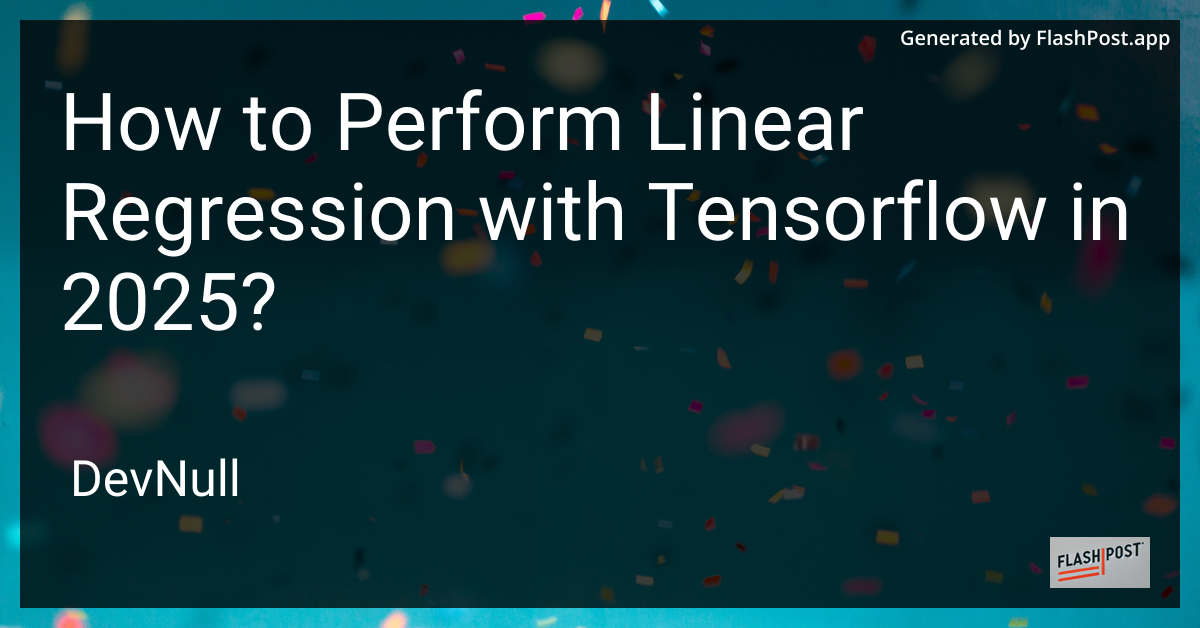
How to Perform Linear Regression with Tensorflow in 2025?
In 2025, TensorFlow continues to be a powerhouse in the realm of machine learning frameworks, offering versatility and ease of use for developing advanced models. Linear regression, one of the foundational algorithms in both statistics and machine learning, remains a key area of study for its simplicity and effectiveness. This guide will walk you through the steps for performing linear regression using TensorFlow, ensuring you leverage the best practices available today.
What is Linear Regression?
Linear regression attempts to model the relationship between two or more variables by fitting a linear equation to observed data. The basic idea is to find a line that best fits the data points, which can then be used to predict outcomes for unseen data.
Setting Up TensorFlow for Linear Regression
Before we perform linear regression, make sure you have TensorFlow installed. You can install it using pip:
pip install tensorflow
Step 1: Import Libraries
Begin by importing the necessary libraries. Apart from TensorFlow, you may need NumPy and Matplotlib for data manipulation and visualization.
import tensorflow as tf
import numpy as np
import matplotlib.pyplot as plt
Step 2: Prepare Your Data
Generate some synthetic data for the purpose of this demonstration. Creating data that fits a linear model helps illustrate how linear regression functions.
x = np.linspace(0, 10, 100)
y = x * 2 + np.random.randn(*x.shape) * 0.5
plt.scatter(x, y)
plt.xlabel('x')
plt.ylabel('y')
plt.title('Data')
plt.show()
Tip: Prior to training any model, it’s crucial to scale your data properly. Learn how to optimize your data for TensorFlow in What is the Right Way to Scale Data for TensorFlow.
Step 3: Define the Linear Regression Model
Using TensorFlow, we’ll set up a simple linear model. TensorFlow provides a straightforward API for building models, including linear regression.
model = tf.keras.Sequential([
tf.keras.layers.Dense(units=1, input_shape=[1])
])
For insights into choosing the correct input shape, you can refer to How to Choose an Input Shape in TensorFlow.
Step 4: Compile the Model
Next, configure the model for training by compiling it with necessary parameters: an optimizer, a loss function, and evaluation metrics.
model.compile(optimizer='sgd', loss='mean_squared_error')
Step 5: Train the Model
Proceed to train the model with your data. This step involves feeding the model with inputs (x) and outputs (y) for it to learn the pattern.
model.fit(x, y, epochs=200)
Step 6: Evaluate and Use the Model
Once trained, you can inspect the model’s performance. TensorFlow models have built-in methods for generating a summary of the architecture, which you can explore here: TensorFlow Model Summary.
Finally, use your model to make predictions.
new_x = np.array([5, 7, 9])
predictions = model.predict(new_x)
print(predictions)
Conclusion
In 2025, TensorFlow streamlines the implementation of linear regression, easing the journey from data preparation to prediction. Its robust and user-friendly APIs make it accessible for both beginners and experts. As you continue your exploration, remember that proper data handling and model configuration are key to optimizing performance.
For further learning, delve into resources on optimizing data scaling and understanding model summaries to ensure that your implementations are efficient and effective.
Happy coding in 2025!