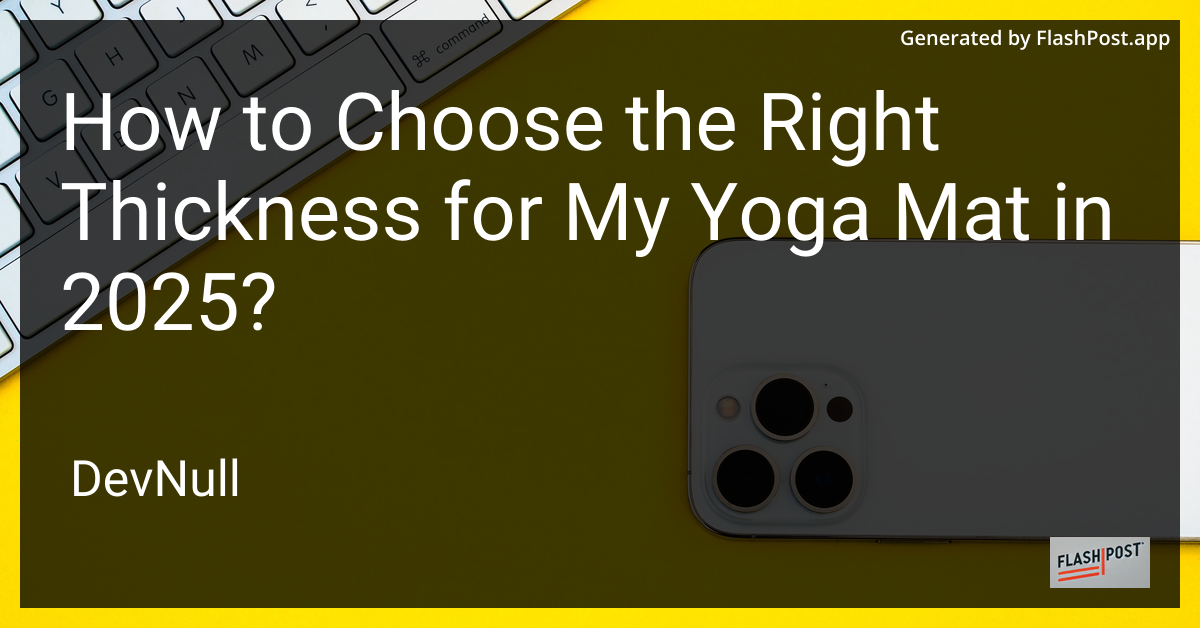
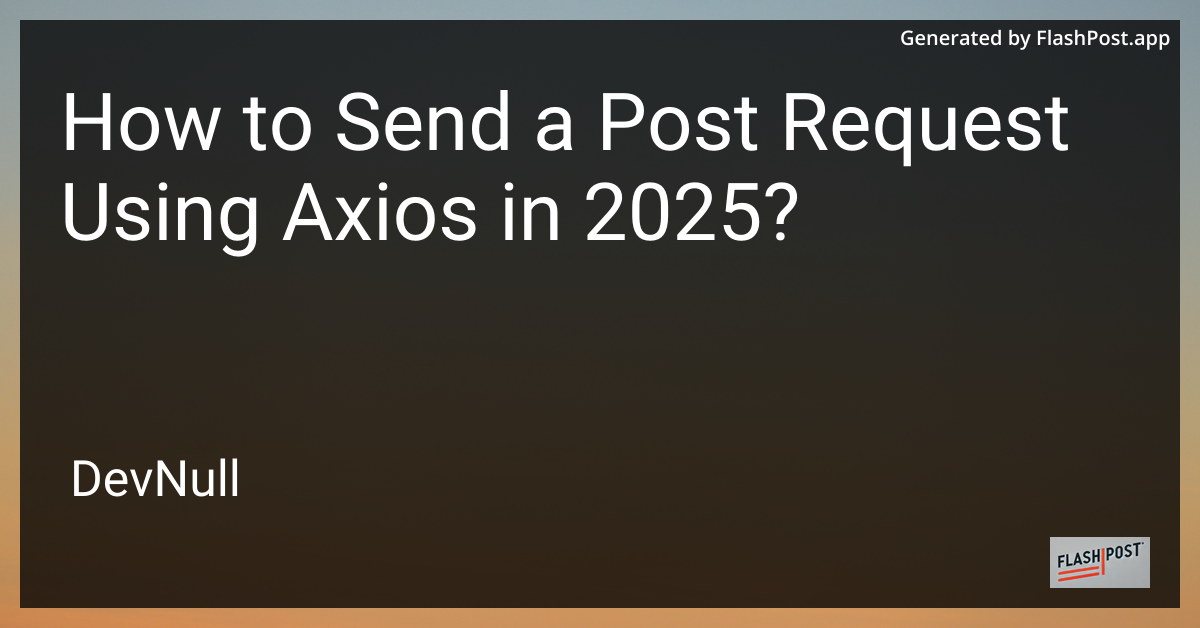
How to Send a Post Request Using Axios in 2025?
In the world of web development, making HTTP requests is a fundamental aspect of client-server communication. Axios, a popular JavaScript library, simplifies the process of making HTTP requests and handling responses in web applications. As we step into 2025, let’s explore how to send a POST request using Axios, ensuring your applications remain modern and efficient.
Introduction to Axios
Axios is a promise-based HTTP client for the browser and Node.js. It provides a simple and clean API for making HTTP requests and handling responses with ease. Axios’ support for older browsers and its rich feature set make it an invaluable tool for developers.
Setting Up Axios
Before you can send a POST request with Axios, you need to have Axios installed in your project. You can do this using npm or yarn:
npm install axios
yarn add axios
Sending a POST Request
In 2025, the fundamental way to send a POST request using Axios hasn’t changed drastically. Here’s a step-by-step guide:
-
Import Axios
Make sure to import Axios at the top of your JavaScript file:
import axios from 'axios';
-
Create the Data Object
Prepare the data you want to send in the POST request. This data is typically in the form of a JavaScript object:
const data = { username: 'yourUsername', password: 'yourPassword' };
-
Send the POST Request
Use the
axios.post
method to send a POST request to a specific URL. Pass the URL and the data object as arguments:axios.post('https://api.example.com/login', data) .then(response => { console.log('Response:', response.data); }) .catch(error => { console.error('Error:', error); });
The
post
method returns a promise. You can handle the response using.then()
and catch errors using.catch()
.
Adding Headers
Sometimes, you need to include additional headers in your request for authentication or content type. You can do this as follows:
axios.post('https://api.example.com/login', data, {
headers: {
'Content-Type': 'application/json',
'Authorization': 'Bearer YOUR_ACCESS_TOKEN'
}
})
.then(response => {
console.log('Response:', response.data);
})
.catch(error => {
console.error('Error:', error);
});
Handling Responses
In 2025, Axios continues to provide robust response handling capabilities. The response object includes data
, status
, statusText
, headers
, and more. You can utilize these properties to handle API responses effectively.
Further Learning
Mastering Axios gives you the edge in handling HTTP requests in modern web applications. Consider broadening your JavaScript expertise with additional resources:
- Explore JavaScript Coding Strategies to enhance your coding techniques.
- Learn how to run JavaScript code over an iframe.
- Discover methods to detect when an iframe is loaded.
Conclusion
Sending a POST request using Axios is a critical skill for any web developer in 2025. By following the steps outlined above, you can efficiently manage HTTP requests and responses in your applications, keeping them seamless and responsive. Happy coding!