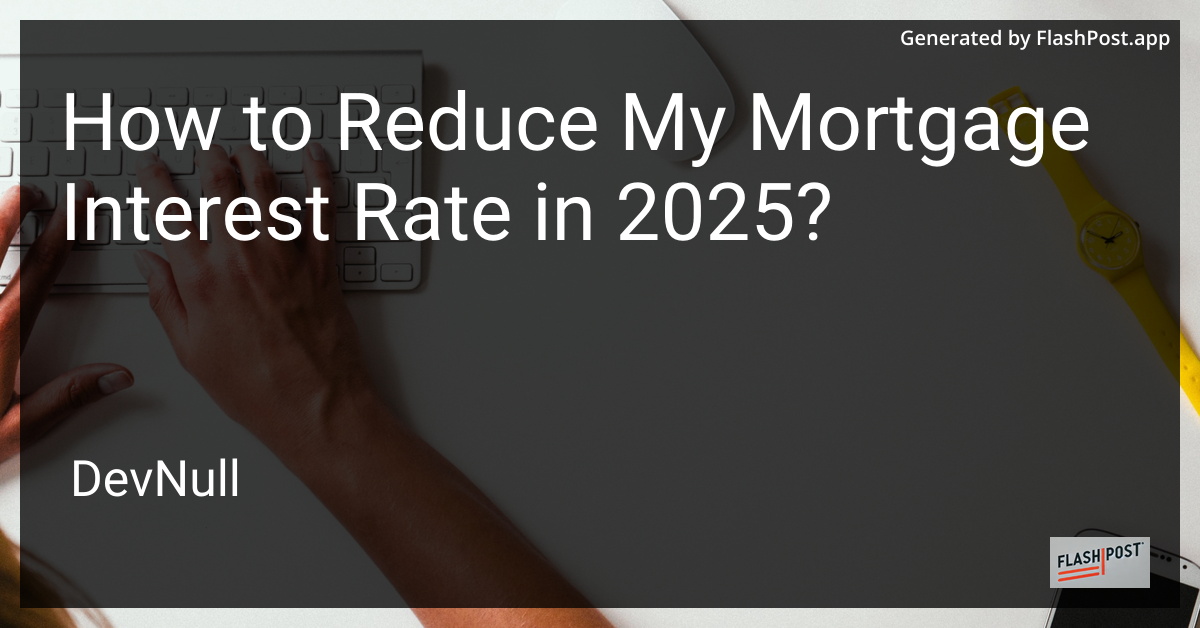
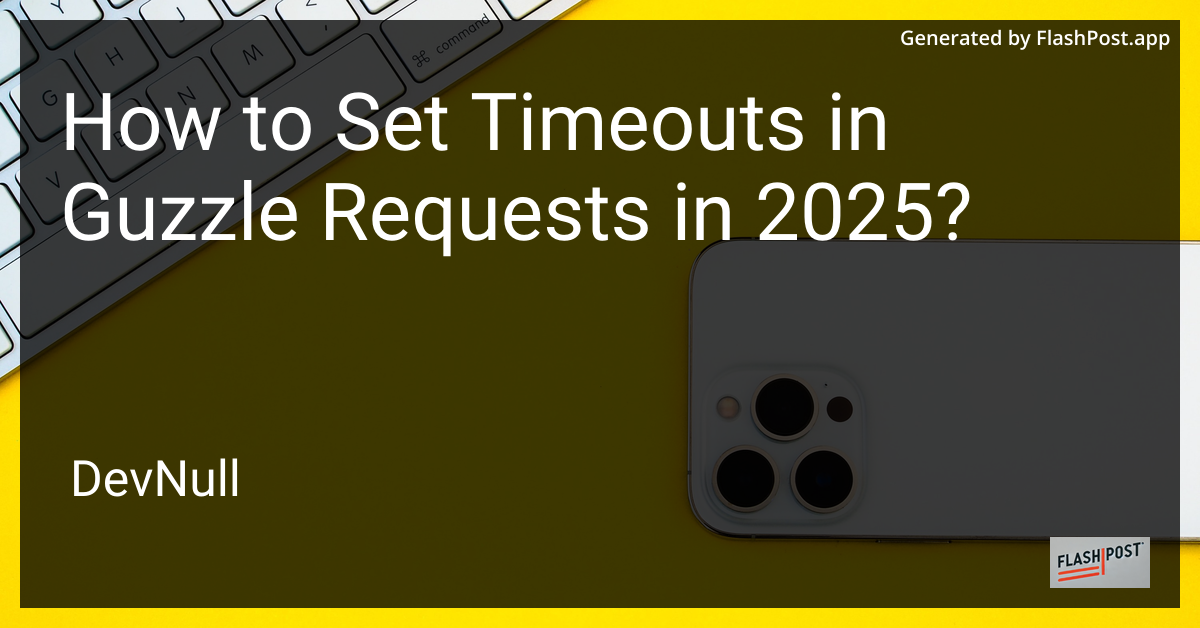
How to Set Timeouts in Guzzle Requests in 2025?
title: How to Set Timeouts in Guzzle Requests in 2025 date: 2025-01-15 author: Tech Enthusiast tags: [Guzzle, Laravel, PHP, HTTP Requests, Timeouts]
Introduction
In 2025, using Guzzle as a PHP HTTP client continues to be a popular choice for developers needing a robust and flexible library. Handling HTTP requests efficiently is crucial, especially when dealing with external APIs that might occasionally slow down or become unresponsive. One essential aspect of managing these requests is setting timeouts to prevent your application from hanging indefinitely.
In this article, we’ll walk through the process of setting timeouts in Guzzle requests, ensuring your Laravel application remains responsive and efficient.
What Are Guzzle Timeouts?
Guzzle timeouts specify the maximum amount of time your request is allowed to wait for a response:
connect_timeout
: Maximum time in seconds the client will wait to establish a connection.timeout
: Maximum time, in seconds, that a client will wait for the entire request to complete, including transfers, after the connection is established.
How to Set Guzzle Timeouts
Setting timeouts in Guzzle is straightforward. When you create a client or send a request, you include the timeout options. Here’s how you can do it:
use GuzzleHttp\Client;
$client = new Client([
'timeout' => 10.0, // Wait a maximum of 10 seconds for the request to complete
'connect_timeout' => 5.0, // Wait a maximum of 5 seconds to connect to the server
]);
try {
$response = $client->request('GET', 'https://api.example.com/data');
echo $response->getBody();
} catch (\GuzzleHttp\Exception\ConnectException $e) {
echo 'Connection timeout: ' . $e->getMessage();
} catch (\GuzzleHttp\Exception\RequestException $e) {
echo 'Request timeout: ' . $e->getMessage();
}
In the snippet above, we’re configuring a Guzzle client with a timeout of 10 seconds and a connection timeout of 5 seconds. This setup helps ensure that your application doesn’t wait too long and becomes unresponsive.
Why Are Timeouts Important?
Timeouts are paramount when dealing with external HTTP requests. Here’s why you should always configure them:
- Prevent Hanging Requests: Avoid having your application stuck waiting for a response that might never come.
- Resource Management: Free up resources for other requests, maintaining overall application performance.
- User Experience: Provide quicker feedback by gracefully handling delays rather than leaving users waiting indefinitely.
Further Learning and Best Practices
- If you are looking for more tailored configurations, especially within Laravel applications, you might find how to disable Guzzle SSL verify in Laravel useful.
- For more complex operations, such as file uploads, check out how to upload file via Guzzle HTTP in Laravel.
- To better understand the Guzzle integration in Laravel, you can explore Laren Guzzle Integration.
Conclusion
Configuring timeouts for Guzzle requests is a necessary practice when managing external HTTP calls. Implement these settings wisely in your Laravel applications to safeguard against potential network issues and optimize the performance and reliability of your applications.
By following the steps outlined in this article, you can ensure your application maintains efficiency and robustness in handling HTTP requests as we progress through 2025 and beyond.
For further reading on Guzzle configurations and integrations, explore the linked resources above that dive deeper into various aspects of Guzzle HTTP client usage within Laravel applications.