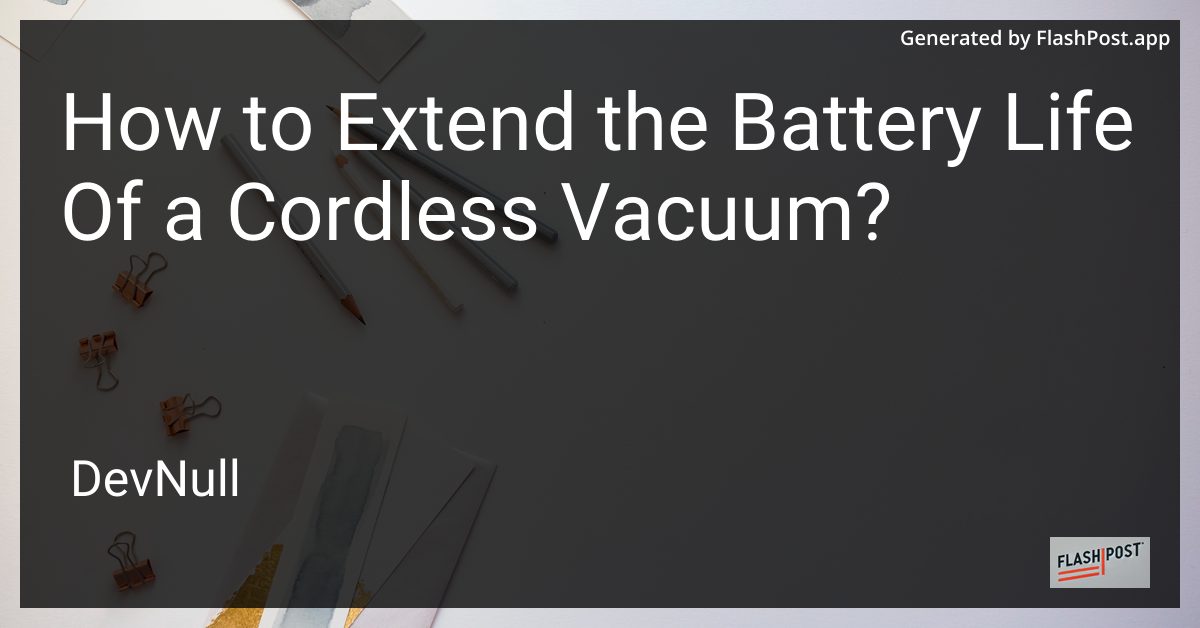
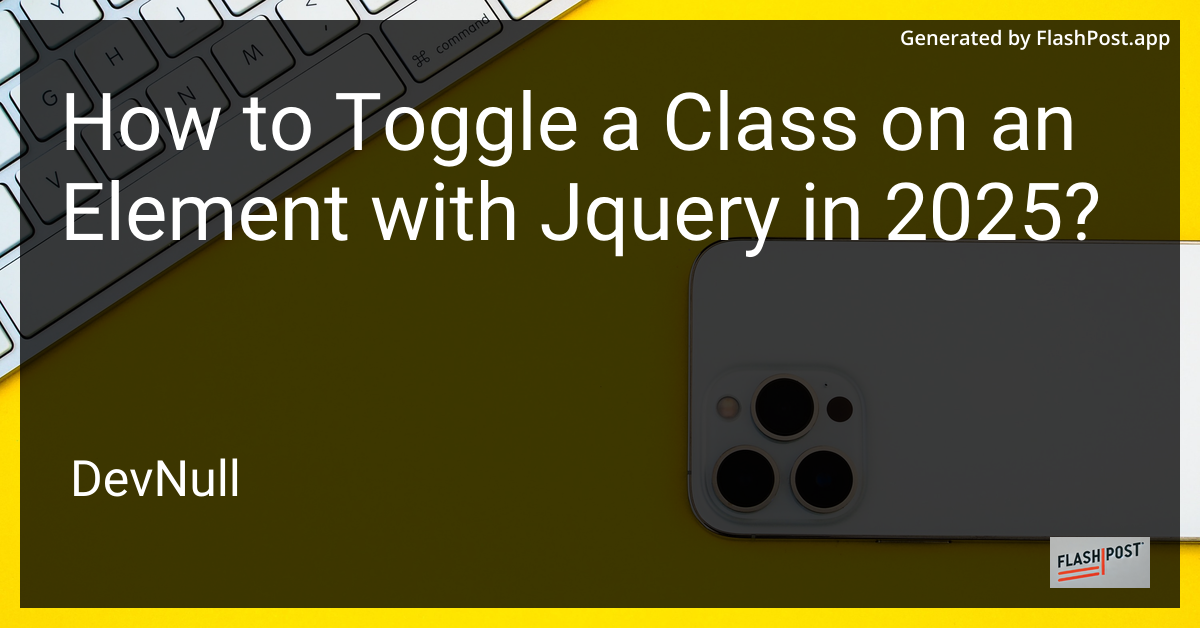
How to Toggle a Class on an Element with Jquery in 2025?
In the ever-evolving world of web development, jQuery continues to hold its ground due to its simplicity and effectiveness. One common task that developers often encounter is toggling a class on an element. This task is straightforward with jQuery. In this article, we will guide you through the steps of toggling a class using jQuery, ensuring your code remains efficient and effective in 2025.
What is Toggling a Class?
Toggling a class involves adding a class to an element if it’s not present and removing it if it is, essentially switching the presence of a class in the element’s class list. This can be highly useful for changing styles dynamically, based on user interactions like clicks or other events.
Why Use jQuery for Class Toggling?
Despite modern frameworks and libraries, jQuery remains a powerful tool due to its concise syntax and ability to simplify complex JavaScript tasks. Its compatibility and ease of use make it ideal for quick implementations and small projects.
Steps to Toggle a Class with jQuery
Step 1: Ensure jQuery is Included
First, make sure jQuery is included in your project. If you are working within specific frameworks, such as Yii2, you can reference this guide on adding jQuery to Yii2.
Step 2: Write the jQuery Code
Below is a basic example of how to toggle a class in jQuery:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Toggle Class Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<style>
.highlight {
background-color: yellow;
}
</style>
</head>
<body>
<button id="toggleButton">Toggle Highlight</button>
<div id="content">This is some content to be highlighted.</div>
<script>
$(document).ready(function(){
$("#toggleButton").click(function(){
$("#content").toggleClass("highlight");
});
});
</script>
</body>
</html>
Explanation of the Code
- HTML Structure: We have a button and a div with some content.
- CSS: The
.highlight
class is defined to change the background color. - JavaScript/jQuery: Upon a button click, the
.toggleClass()
function is invoked to toggle thehighlight
class on the#content
div.
Step 3: Test Your jQuery Code
Testing your jQuery code helps ensure its robustness. Tools like Mocha.js can be utilized to test your scripts. For a complete guide on testing jQuery code, refer to this detailed resource.
Conclusion
In 2025, jQuery still provides a simple and effective way to toggle classes on HTML elements. By following the steps outlined above, you can efficiently manage class toggling in your projects. Whether you’re integrating it into a new or existing project, jQuery makes the implementation straightforward and maintains clarity in your coding process.
For further reading on how to manipulate CSS and classes using jQuery, consider checking out this comprehensive article.
Stay updated with the latest development trends and continue leveraging the power of jQuery in an ever-evolving digital landscape.