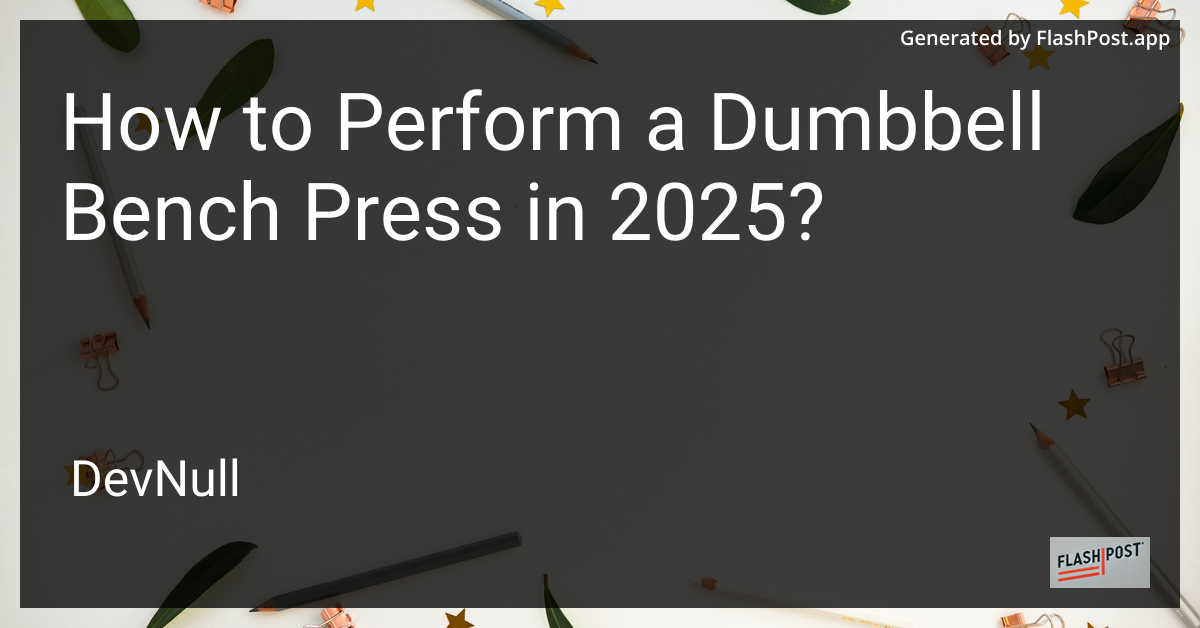
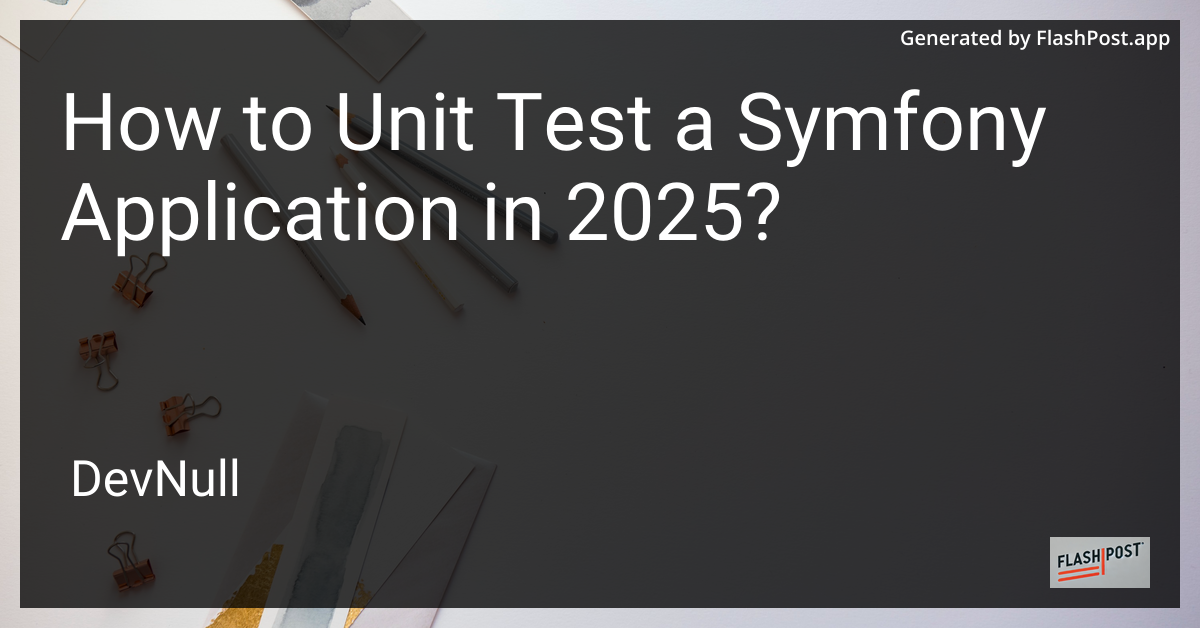
How to Unit Test a Symfony Application in 2025?
Unit testing is an essential practice for maintaining robust and bug-free applications, especially when working with the Symfony framework. As we look towards 2025, here’s an updated guide on how to effectively unit test your Symfony applications using the latest tools and best practices.
Understanding Unit Testing
Unit testing involves testing individual components or functions of an application to ensure they work as intended. In Symfony, unit tests are usually focused on testing services, controllers, and other classes independently, without relying on external integrations.
Setting Up Your Symfony Application for Unit Testing
To start unit testing your Symfony application, ensure you have the following prerequisites in place:
-
Symfony Setup: If you’re setting up Symfony on a Mac, check out this guide on Mac Symfony Setup.
-
Testing Tools: Symfony applications typically use PHPUnit for unit testing. You can install it using Composer:
composer require --dev phpunit/phpunit
-
Configuration: Add a
phpunit.xml
configuration file in your project’s root directory to define how PHPUnit should run your tests.
Writing Unit Tests
-
Create a Test Directory: Organize your tests in the
tests
directory. Symfony follows PSR-4 autoloading, so your test classes should mimic your application’s namespace structure. -
Writing Tests: Create a test file for each class you want to test. Make sure to extend the
TestCase
class provided by PHPUnit.<?php namespace App\Tests\Service; use PHPUnit\Framework\TestCase; use App\Service\YourService; class YourServiceTest extends TestCase { public function testFunctionality() { $service = new YourService(); $result = $service->yourFunction(); $this->assertEquals('expected result', $result); } }
-
Mocking Dependencies: Use PHPUnit’s mocking capabilities to isolate the unit of work by mocking dependencies. This ensures your tests focus only on the functionality of the component in question.
$mockDependency = $this->createMock(SomeDependency::class);
Running Tests
Once your tests are written, you can run them using the following command:
./vendor/bin/phpunit
This will execute all tests in the tests
directory and output the results in your terminal.
Advanced Testing Strategies
As Symfony evolves, so do the testing capabilities. In 2025, take advantage of these strategies:
- Data Providers: For testing multiple data sets with the same logic.
- Test Doubles and Mocks: Advanced object mocking with Prophecy integration.
- CI/CD Integration: Automate testing with Continuous Integration platforms ensuring your tests are run on every commit.
Conclusion
Unit testing is critical for maintaining high-quality Symfony applications. By following these steps, utilizing PHPUnit, and integrating modern testing strategies, you can keep your codebase clean and functional.
For further assistance in building or deploying Symfony applications, check out these resources:
With the right setup and continuous practice, you’ll be able to effectively test and maintain your Symfony applications!