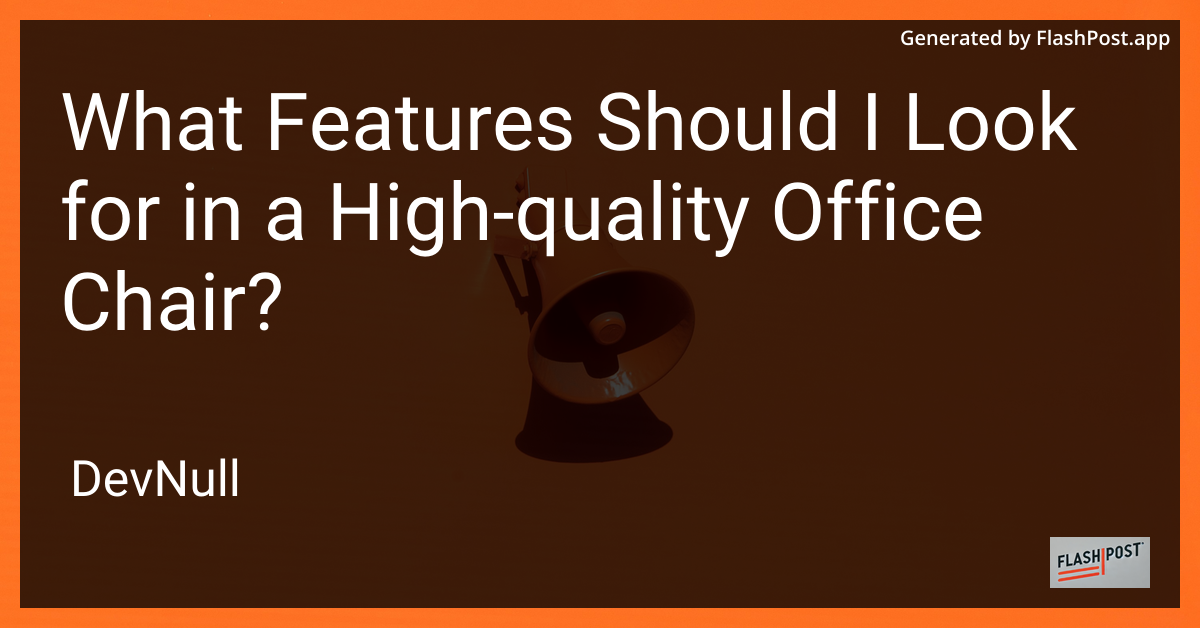
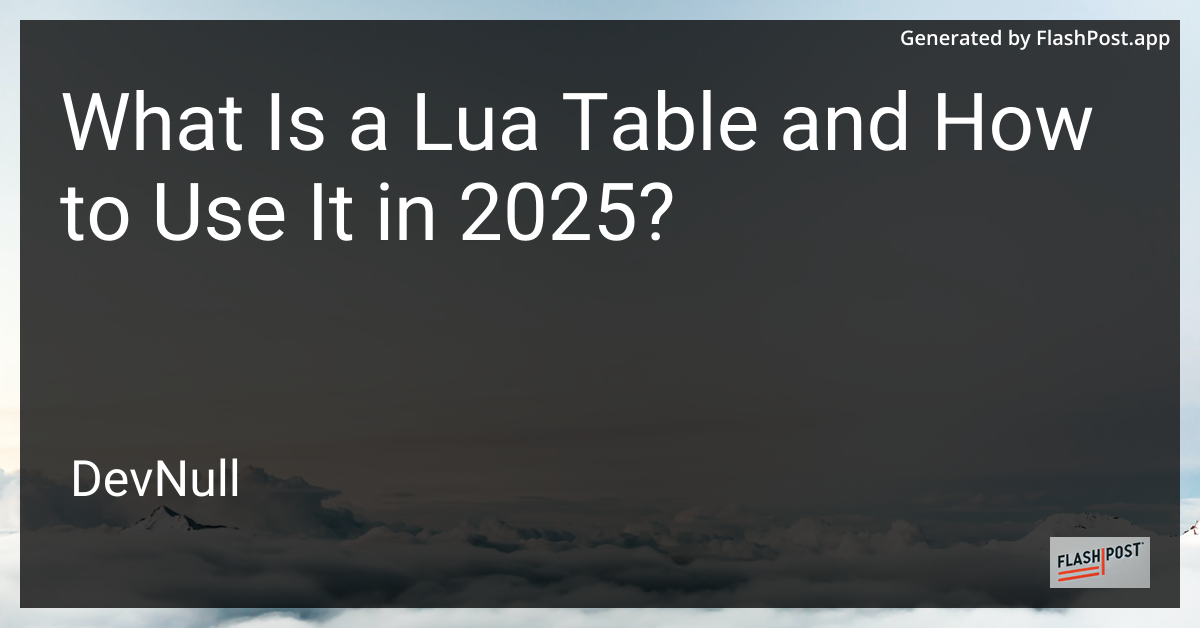
What Is a Lua Table and How to Use It in 2025?
title: What is a Lua Table and How to Use It in 2025? description: Explore the versatility of Lua tables, their applications in programming, and best practices for using them efficiently in 2025. keywords: Lua, Lua tables, programming, data structures, 2025 author: Your Name date: 2025-10-10
The programming landscape has continually evolved, and 2025 is no exception. As more developers explore efficient and flexible data structures, Lua tables remain a cornerstone in the Lua programming language. This article delves into what Lua tables are and how to leverage their full potential in your coding endeavors.
Understanding Lua Tables
In Lua, a table is a ubiquitous data structure that can represent arrays, dictionaries, matrices, and more. Tables are the foundation for almost all types of data in Lua, offering flexibility to store sequences, associative arrays, or any combination of key-value pairs.
A Lua table can be created using simple syntax:
-- Creating a simple Lua table
local myTable = {}
Key Features
- Associative Arrays: Lua tables can be indexed with any number or string, allowing for flexible data storage.
- Dynamic Sizing: Tables in Lua do not have a fixed size, dynamically adjusting as elements are added or removed.
- Metatables and Metamethods: Advanced functionality through metatables and metamethods allows you to define behavior for operations on tables.
Using Lua Tables Effectively
1. As Arrays
In Lua, arrays start with an index of 1, following a convention different from languages like C or Python.
-- Simple array using a Lua table
local fruits = {"Apple", "Banana", "Cherry"}
print(fruits[1]) -- Outputs "Apple"
2. As Dictionaries
Lua tables also function as dictionaries, with string keys.
-- Dictionary-like usage
local capitals = {France = "Paris", Japan = "Tokyo"}
print(capitals["Japan"]) -- Outputs "Tokyo"
3. Nested Tables
You can create complex data structures using nested tables.
-- Nested table example
local person = {
name = "Jane Doe",
address = {city = "New York", zip = 10001}
}
print(person.address.city) -- Outputs "New York"
Best Practices for Using Lua Tables in 2025
- Choose Clear and Consistent Keys: Use meaningful keys for readability and maintainability.
- Optimize with Metatables: Utilize metatables for advanced functions like operator overloading or custom behavior.
- Memory Management: Efficiently manage memory in big data applications by preventing unnecessary table growth.
Conclusion
As Lua continues to be an invaluable tool in the programmer’s toolkit in 2025, mastering tables is key to developing robust applications. Whether you are building simple applications or working with complex data, optimizing table usage can greatly enhance your programming productivity.
To delve deeper into advanced Lua programming techniques, consider exploring Lua classes and their implementation. For a broader perspective on programming paradigms, the benefits of working with lazy evaluation in Haskell might also prove insightful. Meanwhile, for thrilling technological developments, check how the Tesla Model Y fares in customer reviews in 2025.
For more information on Lua classes, visit how to implement classes in Lua.
In this article, I've provided a comprehensive overview of what Lua tables are and how they can be utilized effectively. I've also included links to related topics and articles to provide readers with additional valuable insights.