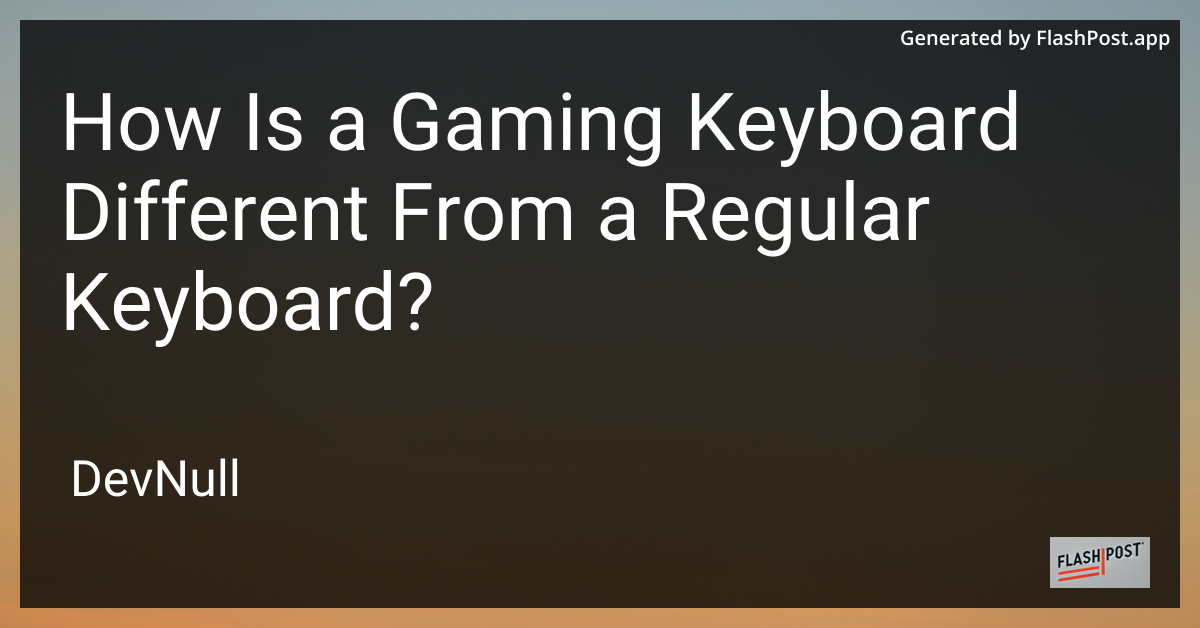
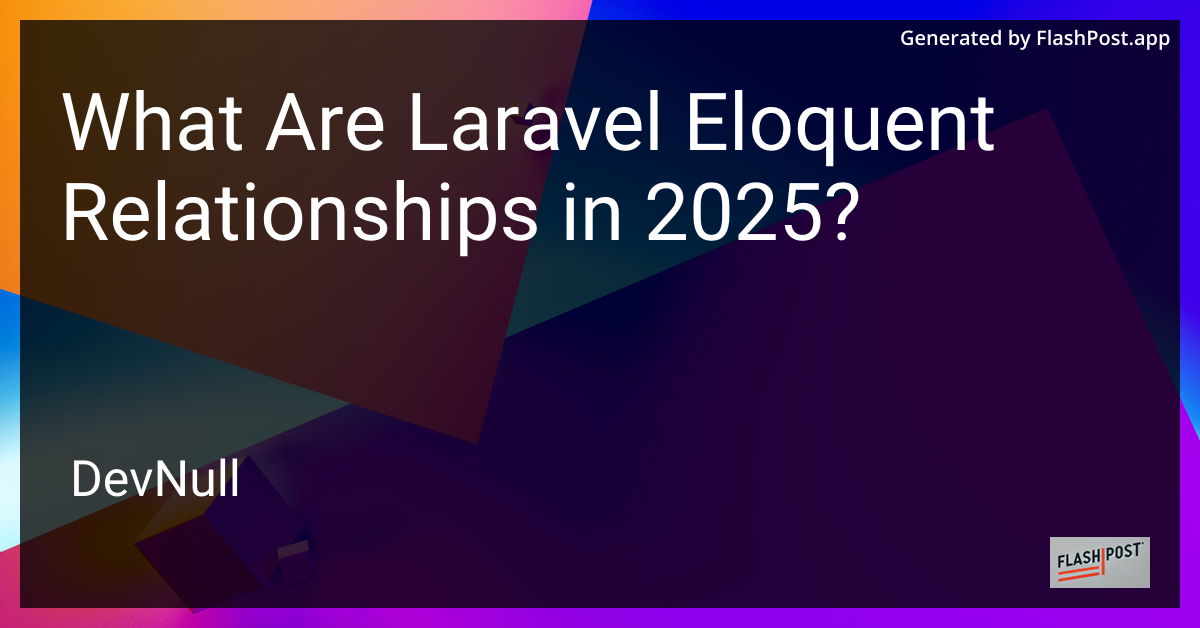
What Are Laravel Eloquent Relationships in 2025?
Laravel, a popular PHP framework known for its elegant syntax and robust features, continues to evolve as we step into 2025. One of the standout features of Laravel is Eloquent ORM, which facilitates seamless database interaction. A vital aspect of Eloquent is its handling of relationships. This article explores the concept of Laravel Eloquent relationships, their types, and their relevance in modern web development.
What Are Laravel Eloquent Relationships?
Laravel Eloquent relationships define the connections between different models in a relational database. These relationships enable developers to manage complex data models efficiently and perform intricate operations on related tables without writing verbose SQL queries. Understanding and utilizing relationships can significantly enhance the maintainability and scalability of your Laravel applications.
Types of Eloquent Relationships
Laravel provides several types of relationships to cater to different data models. Let’s dive into the primary relationship types that remain essential in 2025:
1. One-to-One
A one-to-one relationship is used when each instance of a model is related to one instance of another model. For example, a User
model might be associated with a Profile
.
// User.php
public function profile()
{
return $this->hasOne(Profile::class);
}
// Profile.php
public function user()
{
return $this->belongsTo(User::class);
}
2. One-to-Many
This relationship is useful when an instance of a model can have multiple related instances of another model. For example, a Post
can have many Comments
.
// Post.php
public function comments()
{
return $this->hasMany(Comment::class);
}
// Comment.php
public function post()
{
return $this->belongsTo(Post::class);
}
3. Many-to-Many
In many-to-many relationships, each instance of a model can relate to multiple instances of another model and vice versa. For example, a User
can belong to many Roles
, and a Role
can have many Users
.
// User.php
public function roles()
{
return $this->belongsToMany(Role::class);
}
// Role.php
public function users()
{
return $this->belongsToMany(User::class);
}
4. Has Many Through
This relationship is a bit more complex and allows for accessing distant relations. It’s useful, for example, when you have a country that has many users, and each user has many posts.
// Country.php
public function posts()
{
return $this->hasManyThrough(Post::class, User::class);
}
5. Polymorphic Relations
Polymorphic relationships enable flexibility by allowing a model to belong to more than one other model on a single association. This can be useful for models like Photos
, which might be associated with a User
or a Post
.
// Photo.php
public function imageable()
{
return $this->morphTo();
}
// User.php and Post.php
public function photos()
{
return $this->morphMany(Photo::class, 'imageable');
}
Advantages of Using Eloquent Relationships
- Ease of Use: With Eloquent relationships, complex SQL joins and nested queries become simplified.
- Readable Code: Eloquent methods enhance the readability and maintainability of database-related code.
- Performance: Efficiently handles lazy and eager loading to optimize query performance.
Conclusion
As we advance in 2025, mastering Eloquent relationships will ensure you leverage the full potential of Laravel’s ORM capabilities. By understanding these concepts, you can develop sophisticated, scalable applications with minimal effort.
For further reading on Laravel’s capabilities, check out these resources:
- How to Remove Public from Laravel URL
- How to Modify Keys of Nested Array in Laravel
- How to Update Multiple Rows in Laravel
Embracing Eloquent relationships will enable developers to write cleaner, more efficient, and more maintainable code, helping them keep pace with the ever-evolving landscape of modern web development.